Background
Creating and updating content can be daunting. We use the OpenAI API to generate human-like text for websites and other platforms. This speeds our process up and allows us to produce more content for marketing, research, and SEO purposes.
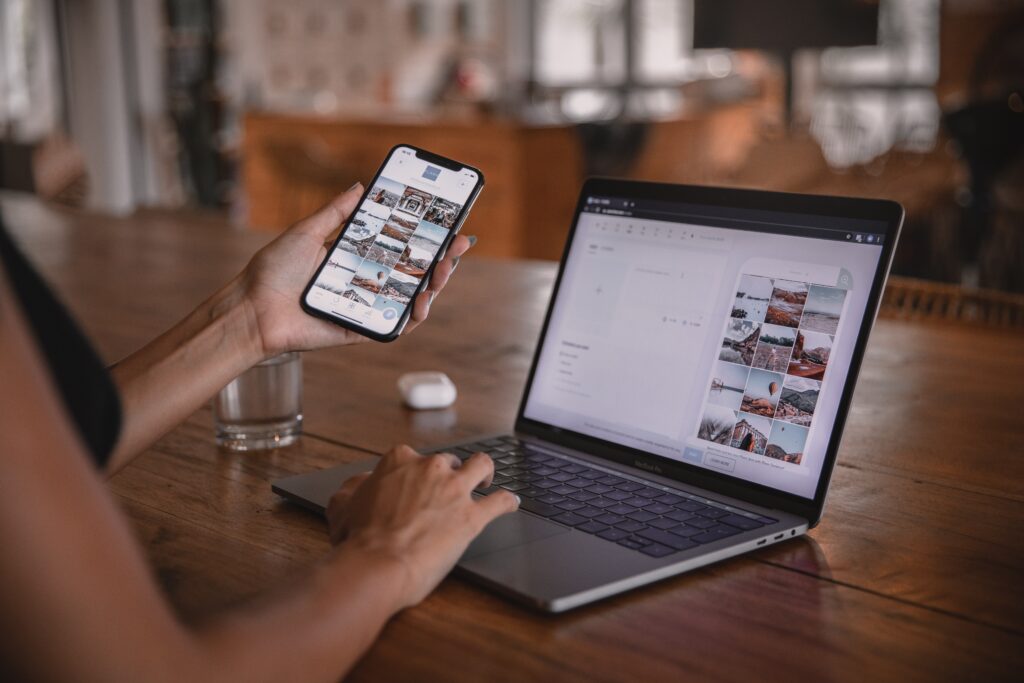
This comes in handy for agencies working on several websites at once. Or any small business looking to improve its website’s SEO by publishing more SEO-friendly pages and posts.
Some ways we currently use this tool:
- Daily social media and Google Business posts get generated automatically
- Generate blog ideas for a specific website
- Generate full-text blog posts optimized for SEO
- Review existing website and run automatically audits
This has given us the opportunity to generate websites at scale.
CODE Examples
Get all the pages for a website by scrapping its sitemap.
import requests
from xml.etree import ElementTree as ET
def get_sitemap_links(sitemap_url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537',
'Accept': 'text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8'
}
response = requests.get(sitemap_url, headers=headers)
response.raise_for_status()
tree = ET.fromstring(response.content)
ns = {'sitemap': 'http://www.sitemaps.org/schemas/sitemap/0.9'}
urls = [url.text for url in tree.findall('.//sitemap:loc', namespaces=ns)]
return urls
def main():
print("Welcome to Octopi's Sitemap URL Extractor!")
sitemap_url = input("Please enter the sitemap URL: ")
try:
links = get_sitemap_links(sitemap_url)
if not links:
print("No URLs found in the sitemap.")
return
print("\nExtracted URLs:")
for link in links:
print(link)
except requests.RequestException as e:
print(f"Error: {e}")
if __name__ == "__main__":
main()
Make a request to the OpenAI API to generate blog titles
text = openai.Completion.create(
model="text-davinci-003",
prompt='''
IAC services Connecticut with professional air duct cleaning and indoor air care services. We Service The Following Connecticut Areas With Professional Air Duct Cleaning: Hartford, New Haven, Stamford,
You just got hired as a skilled content writer for IAC . You're first job is to provide 20 blog ideas about this research.
The focus on these blog posts to improve the website's ranking for "air duct cleaning" "HVAC cleaning" and "dryer vent cleaning" searches for Local SEO in the towns listed above.
Return it in a list, without numbers and seperate them with commas. Make them random in order. The list needs to be in a python format list.
''',
max_tokens=1000,
temperature=0.7,
top_p=1
)
Now you can get quality blog titles for a website. You can then write them yourself and use more creative prompts to generate full text blog posts, optimized for SEO, and tailored specifically to your business.